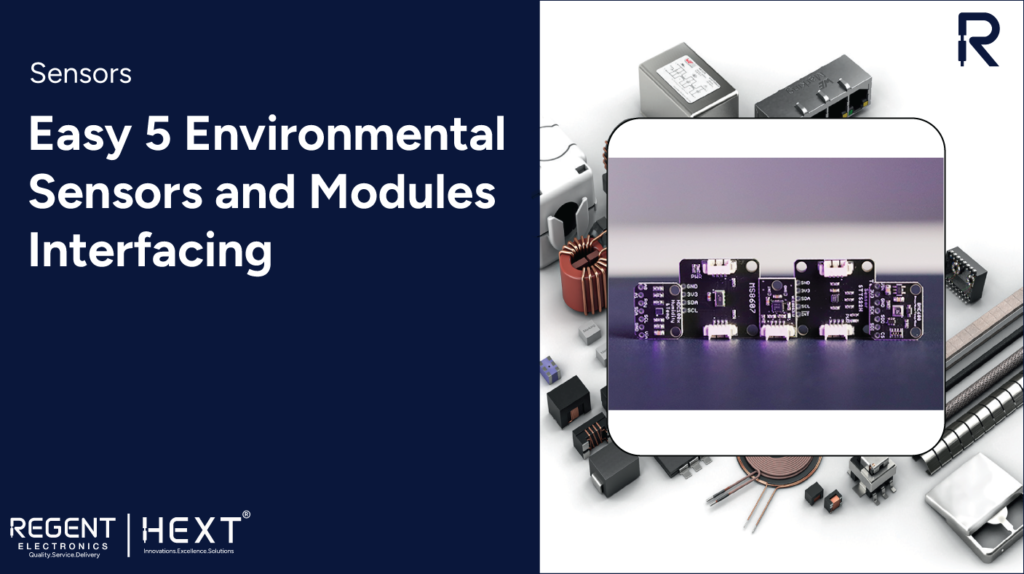
Easy Regent Electronics 5 Environmental Sensors and Modules Interfacing
Introduction
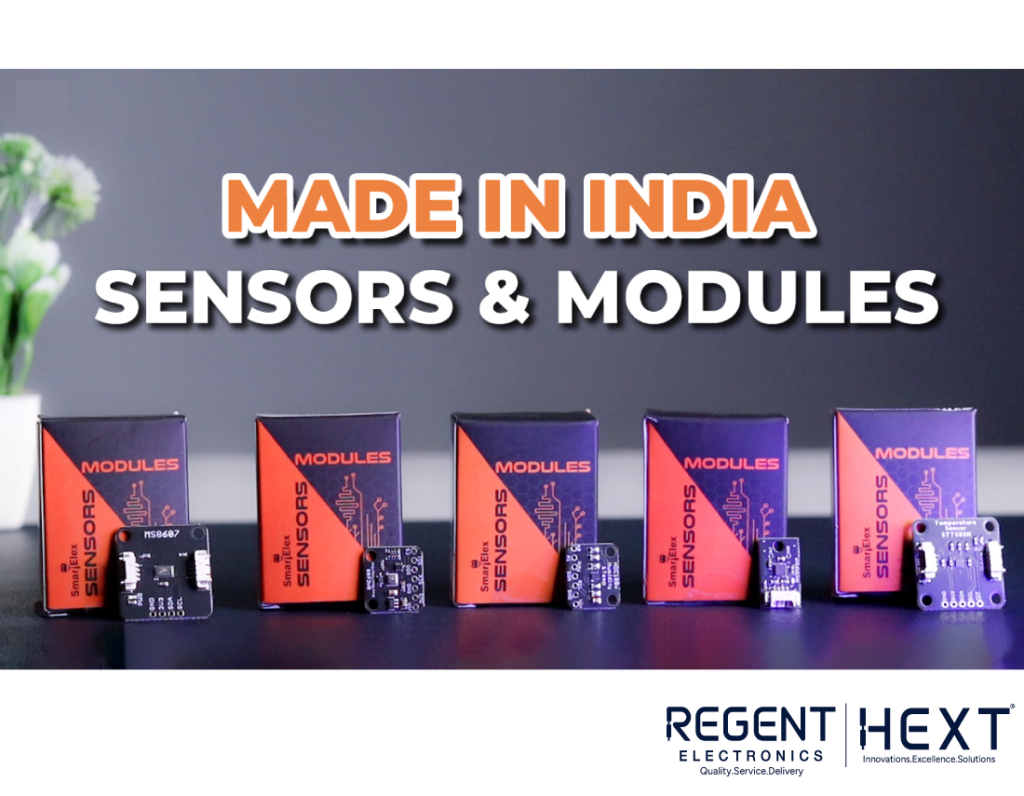
Welcome to the world of smart digital environmental sensors! At Regent Electronics, we offer high-quality temperature, humidity, pressure, weather, and magnetometer sensors. In this guide, we will compare these sensors with generic alternatives and demonstrate how to interface them with an Arduino.
Topics Covered:
- Understanding Environmental Sensors
- Required Hardware and Software
- Temperature Sensor and Its Code
- Humidity Sensor and Its Code
- Pressure Sensor and Its Code
- Weather Sensor and Its Code
- Magnetic Sensor and Its Code
- Results and Conclusion
Understanding Environmental Sensors
Environmental sensors measure parameters like temperature, humidity, pressure, and magnetic fields, essential for IoT and industrial applications. They provide accurate real-time data for monitoring environmental conditions effectively.
Required Hardware
- Regent Electronics Temperature Sensor
- Regent Electronics Humidity Sensor
- Regent Electronics Pressure Sensor
- Regent Electronics Weather Sensor
- Regent Electronics Magnetometer Sensor
- Arduino Uno with Cable
- Jumper Wires
- Breadboard
Required Software
- Arduino IDE
Temperature Sensor (STTS22H)
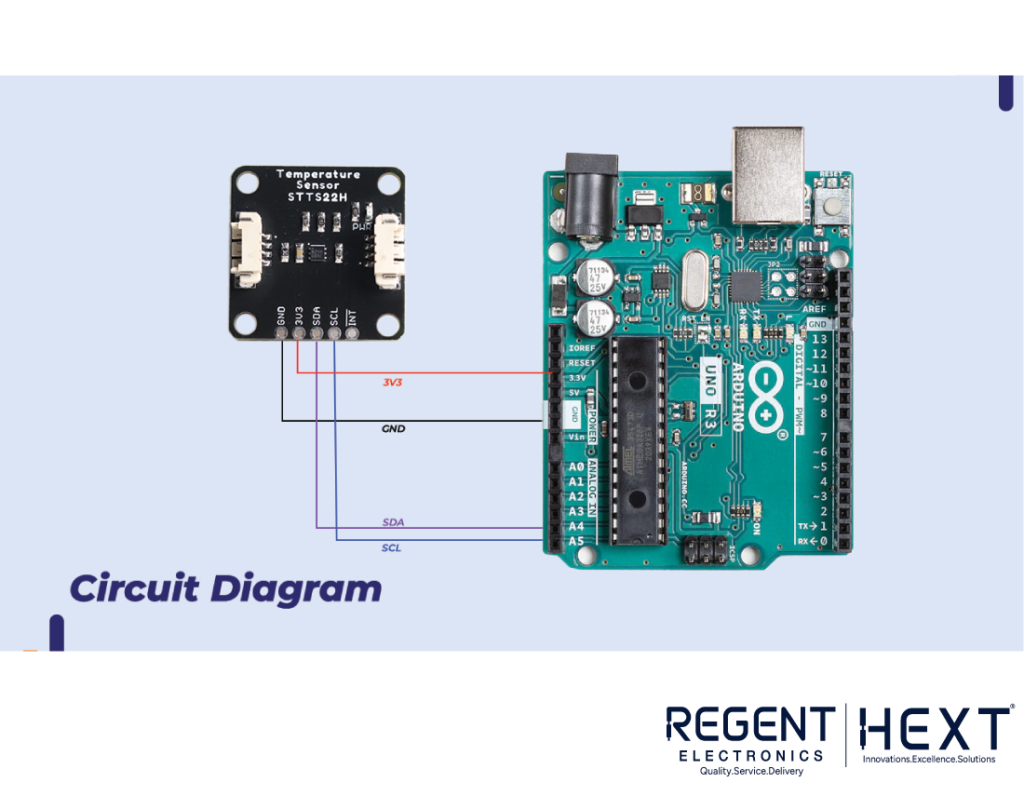
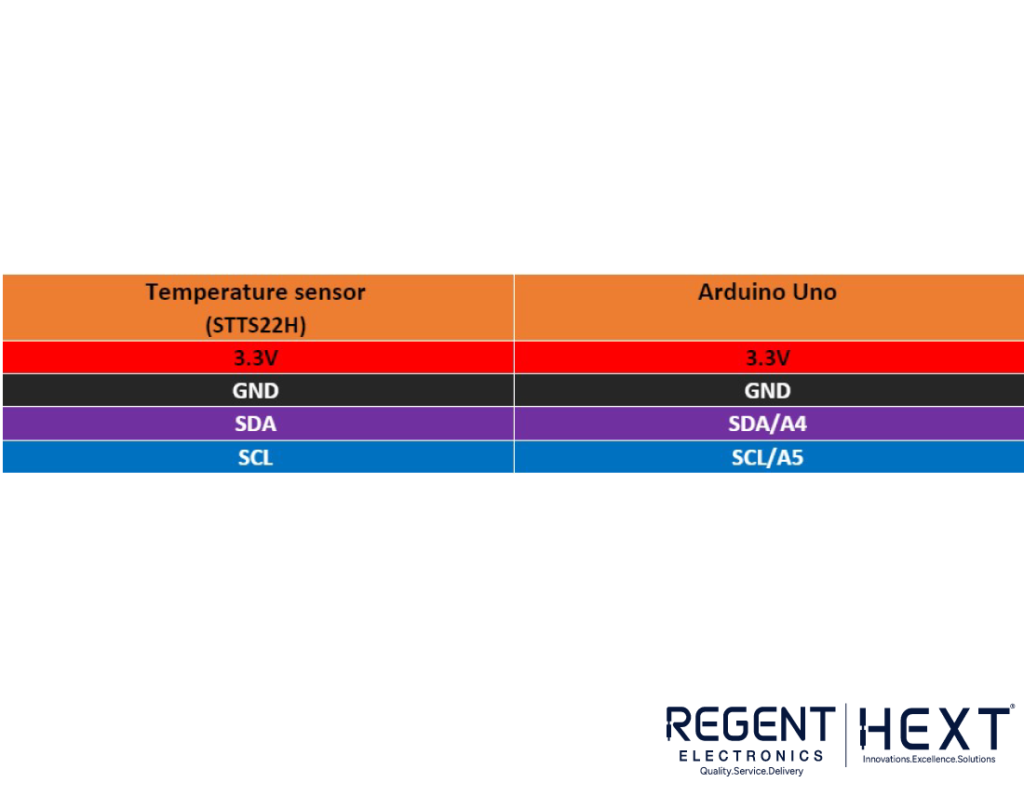
Features:
- I2C interface with multiple address options
- Temperature range: -40°C to +125°C
- Accuracy: ±0.5°C (-10°C to +60°C)
- Low power consumption (1.75µA in one-shot mode)
Code for Temperature Sensor:
#include <Wire.h>
#include “SparkFun_STTS22H.h”
SparkFun_STTS22H tempSensor;
float tempF, tempC;
void setup() {
Wire.begin();
Serial.begin(115200);
if (!tempSensor.begin()) {
Serial.println(“Sensor initialization failed”);
while (1);
}
Serial.println(“Sensor Ready”);
tempSensor.setDataRate(STTS22H_1Hz);
tempSensor.enableAutoIncrement();
}
void loop() {
if (tempSensor.dataReady()) {
tempSensor.getTemperatureF(&tempF);
tempC = (tempF – 32) * 5 / 9;
Serial.print(“Temp (F): “); Serial.print(tempF);
Serial.print(” Temp (C): “); Serial.println(tempC);
}
delay(1000);
}
Humidity Sensor (HDC100X)
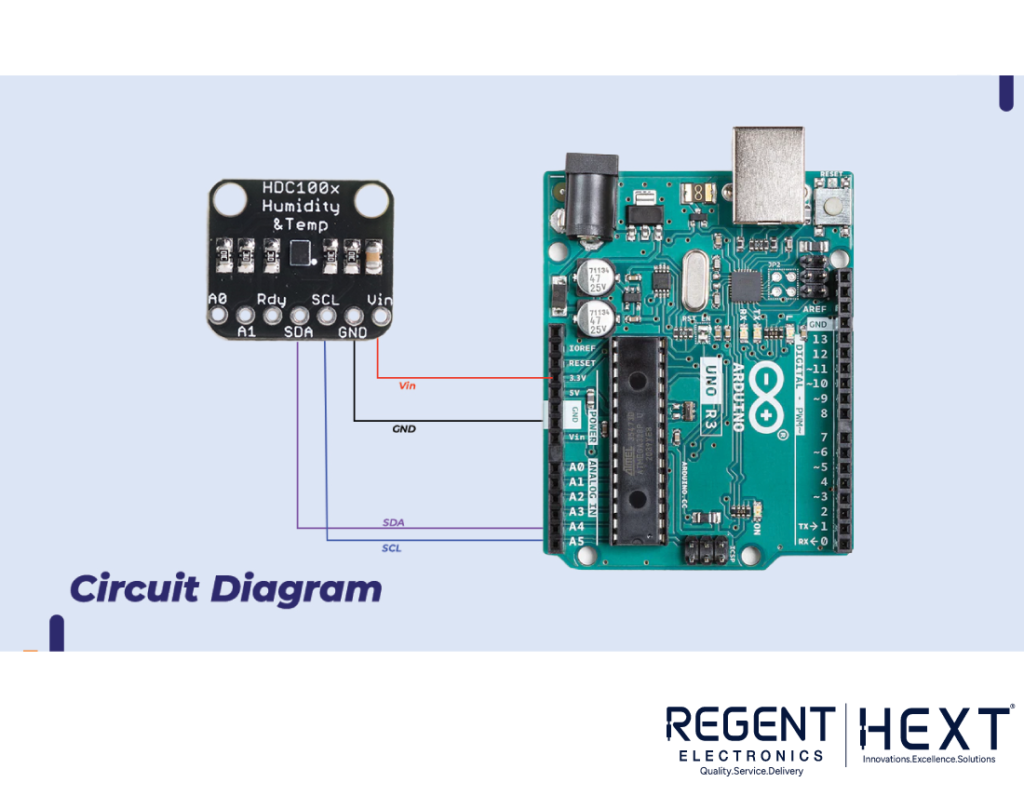
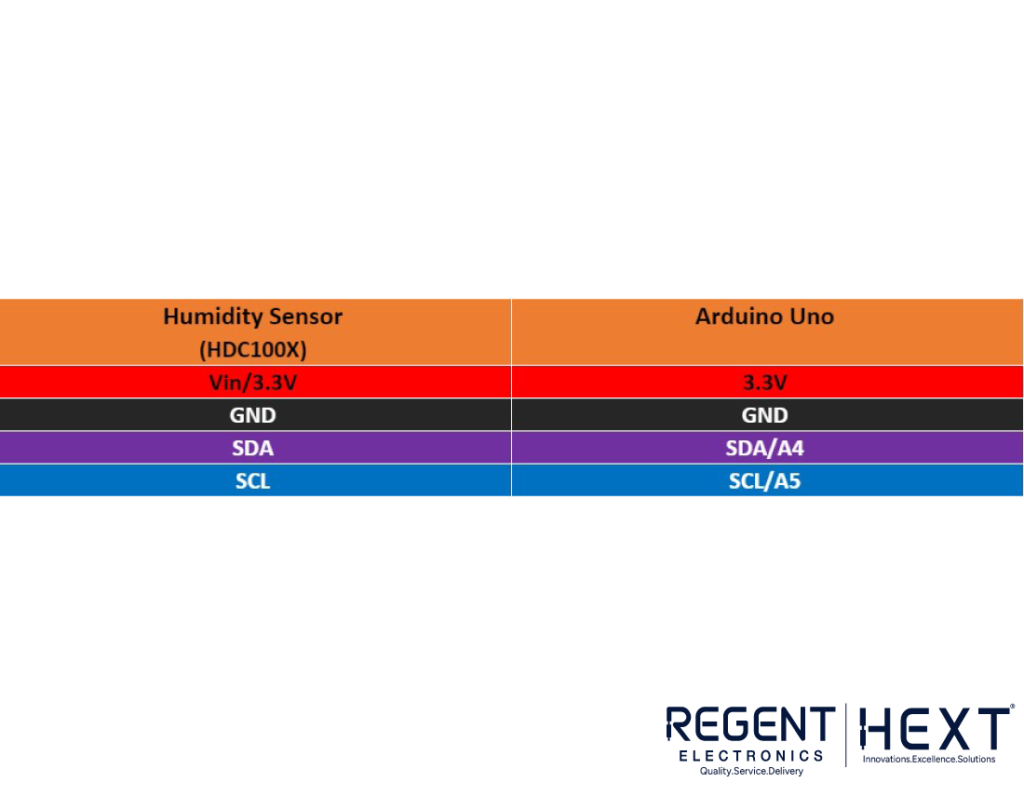
Features:
- Measures both temperature and humidity
- RH Operating Range: 0% to 100%
- Accuracy: ±0.2°C for temperature, ±3% for humidity
- I2C interface
Code for Humidity Sensor:
#include <Wire.h>
#include “Adafruit_HDC1000.h”
Adafruit_HDC1000 hdc;
void setup() {
Serial.begin(9600);
if (!hdc.begin()) {
Serial.println(“Sensor not found!”);
while (1);
}
}
void loop() {
Serial.print(“Temp: “); Serial.print(hdc.readTemperature());
Serial.print(” Hum: “); Serial.println(hdc.readHumidity());
delay(500);
}
Pressure Sensor (BME680)
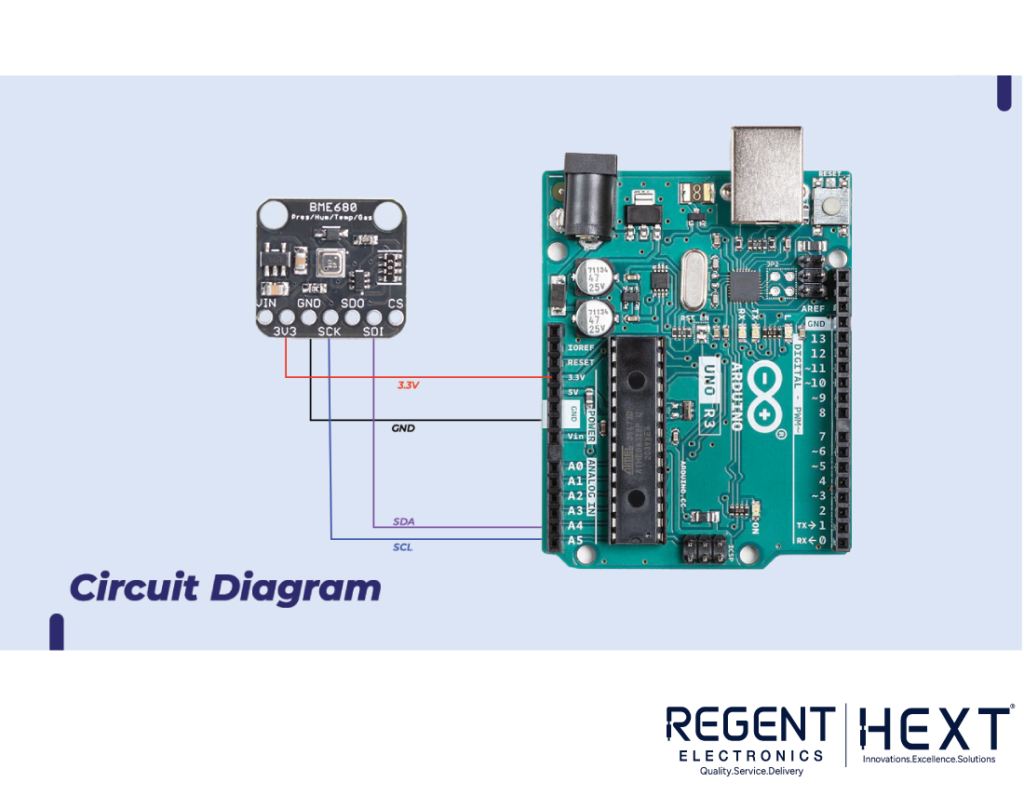
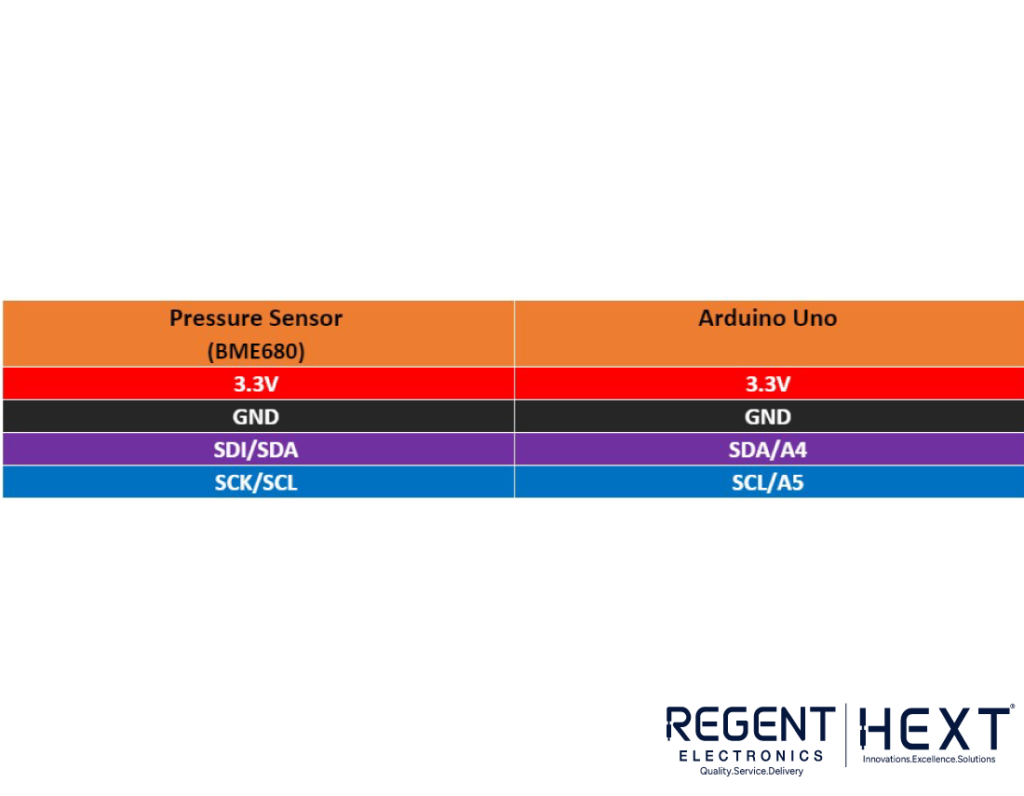
Features:
- Operating voltage: 1.71V to 3.6V
- Humidity Accuracy: ±3%RH
- Pressure Accuracy: ±60Pa
- Temperature Accuracy: ±0.5°C
Code for Pressure Sensor:
#include <Wire.h>
#include “Adafruit_BME680.h”
Adafruit_BME680 bme;
void setup() {
Serial.begin(9600);
if (!bme.begin()) {
Serial.println(“Sensor not found!”);
while (1);
}
}
void loop() {
if (bme.performReading()) {
Serial.print(“Temperature: “); Serial.print(bme.temperature); Serial.println(” *C”);
Serial.print(“Pressure: “); Serial.print(bme.pressure / 100.0); Serial.println(” hPa”);
}
delay(2000);
}
Weather Sensor (MS8607)
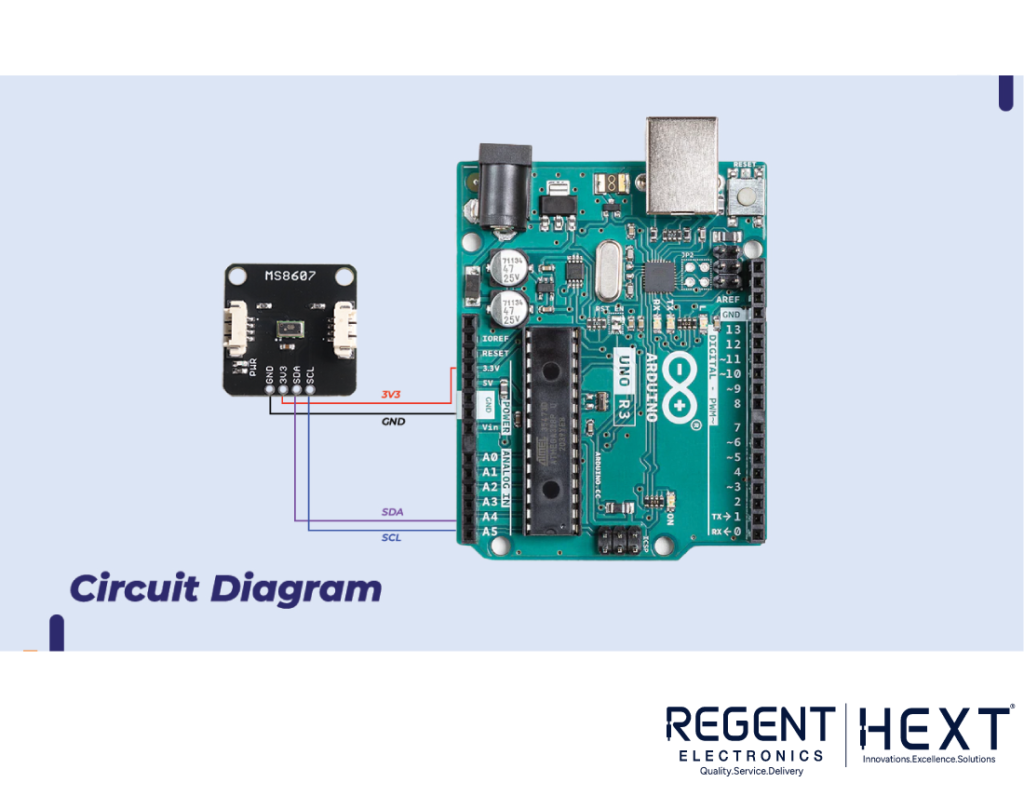
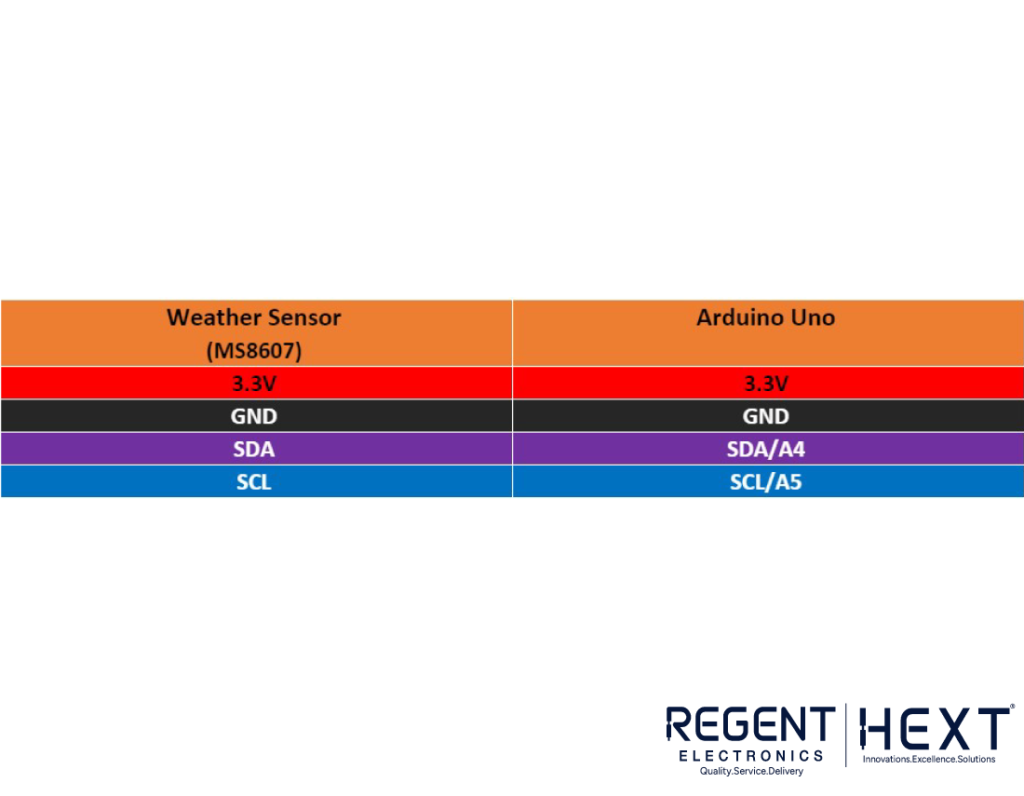
Features:
- Pressure range: 10–2000 mbar
- Humidity range: 0% to 100%
- Temperature range: -40°C to +85°C
Code for Weather Sensor:
#include <Wire.h>
#include “Adafruit_MS8607.h”
Adafruit_MS8607 ms8607;
void setup() {
Serial.begin(115200);
if (!ms8607.begin()) {
Serial.println(“Sensor initialization failed”);
while (1);
}
}
void loop() {
sensors_event_t temp, pressure, humidity;
ms8607.getEvent(&pressure, &temp, &humidity);
Serial.print(“Temperature: “); Serial.println(temp.temperature);
Serial.print(“Pressure: “); Serial.println(pressure.pressure);
Serial.print(“Humidity: “); Serial.println(humidity.relative_humidity);
delay(500);
}
Magnetic Sensor (MMC5983MA)
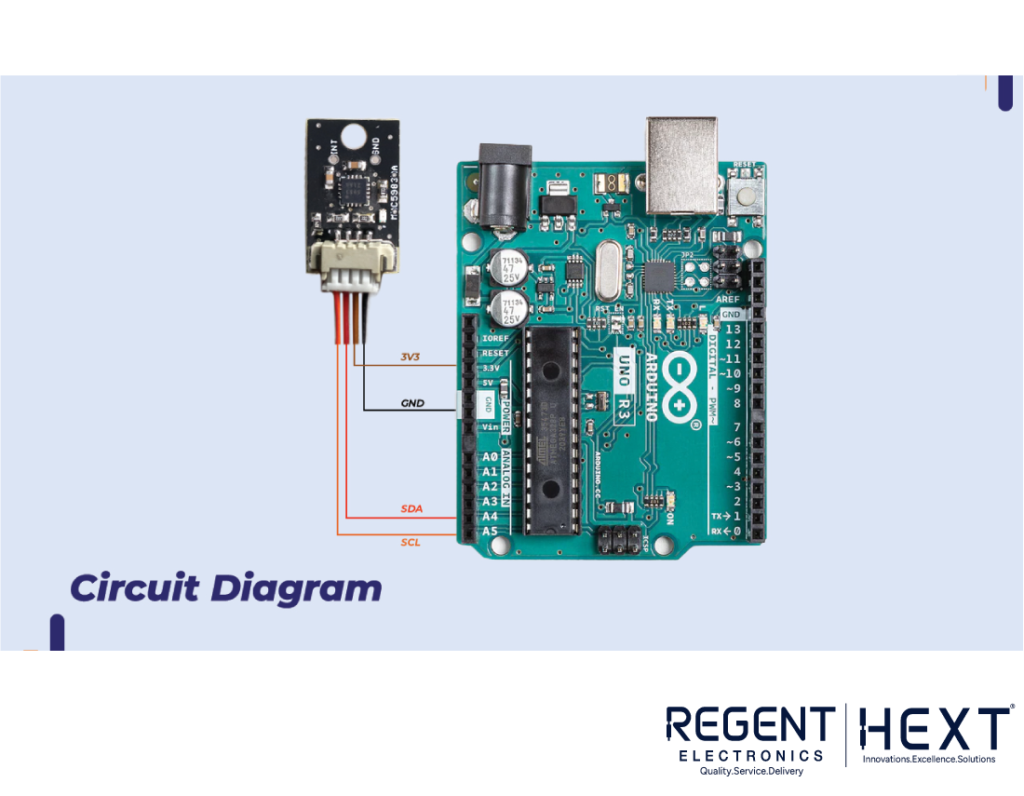
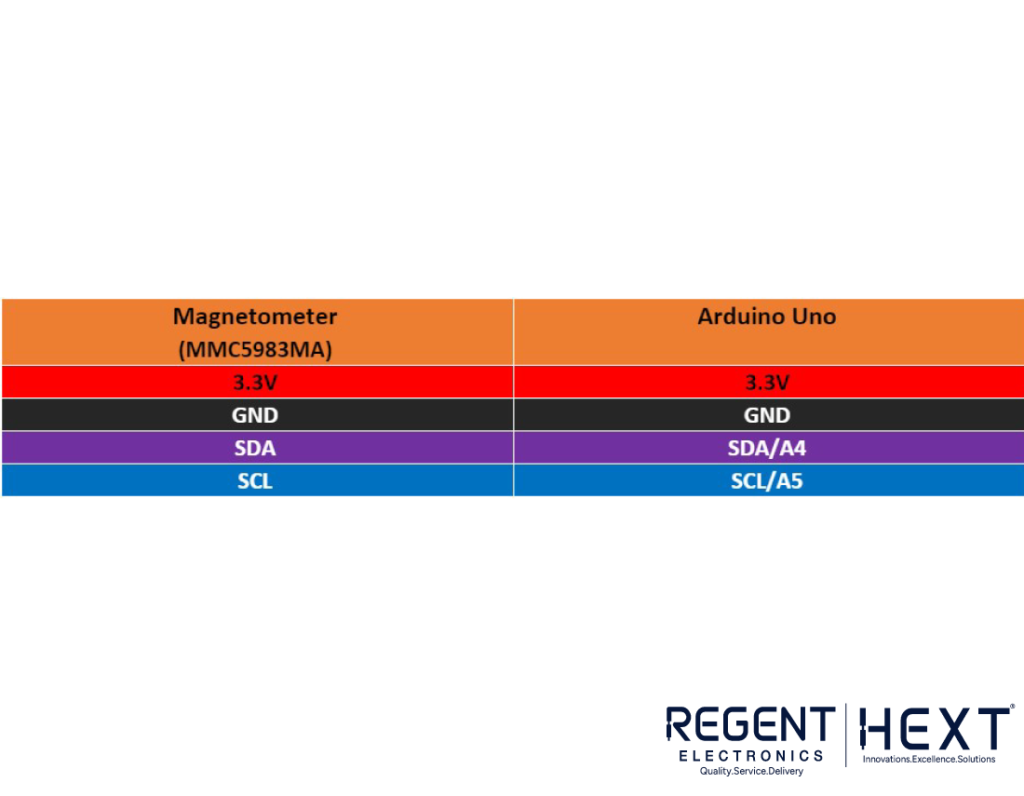
Features:
- Three-axis magnetic field detection
- High accuracy with ±0.5° heading accuracy
- I2C interface
Code for Magnetometer Sensor:
#include <Wire.h>
#include “SparkFun_MMC5983MA_Arduino_Library.h”
SFE_MMC5983MA myMag;
void setup() {
Serial.begin(115200);
Wire.begin();
if (!myMag.begin()) {
Serial.println(“Sensor initialization failed”);
while (1);
}
}
void loop() {
double heading = atan2(myMag.getMeasurementX(), -myMag.getMeasurementY()) * 180 / PI + 360;
Serial.print(“Heading: “); Serial.println(heading, 1);
delay(100);
}
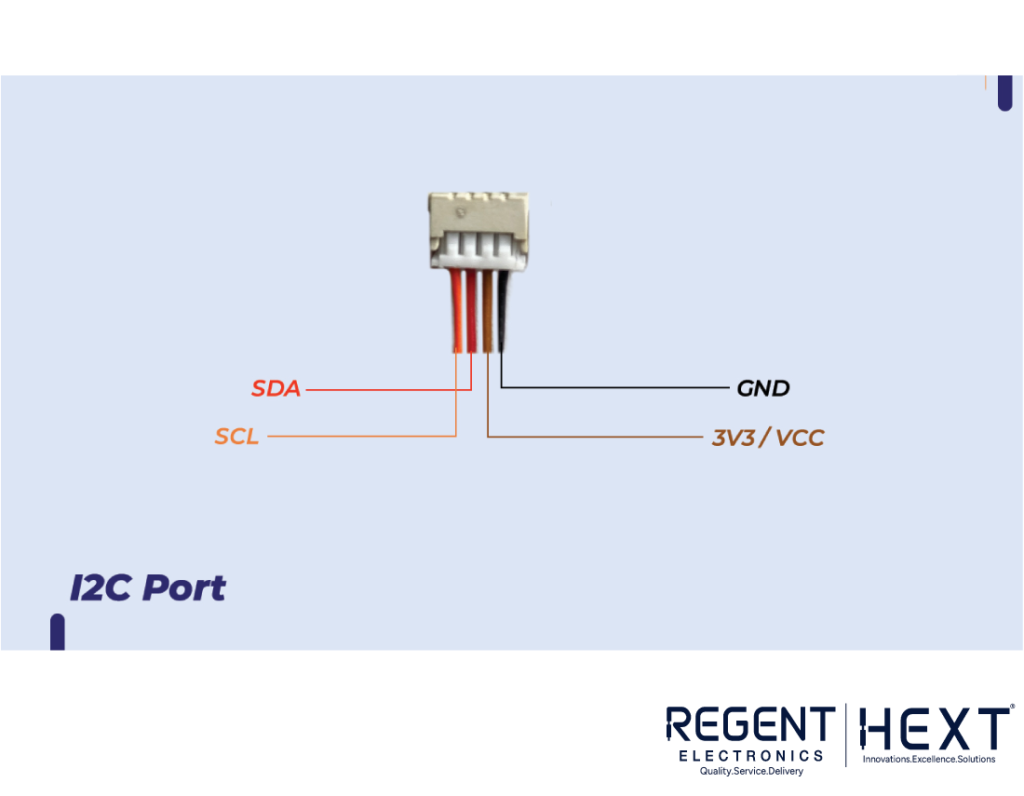
Results and Conclusion
We successfully interfaced Regent Electronics sensors with an Arduino and compared them to generic alternatives like DHT22, BMP180, and HMC5883L. Regent Electronics sensors consistently outperformed the competition in accuracy, reliability, and ease of integration.
Why Choose Regent Electronics Sensors?
- Superior Accuracy: Ensures precise environmental monitoring
- High Reliability: Ideal for industrial and IoT applications
- Easy Integration: Seamless compatibility with Arduino and other microcontrollers
Regent Electronics provides industry-leading environmental sensors, making them the perfect choice for high-precision applications in IoT, industrial automation, and scientific research.