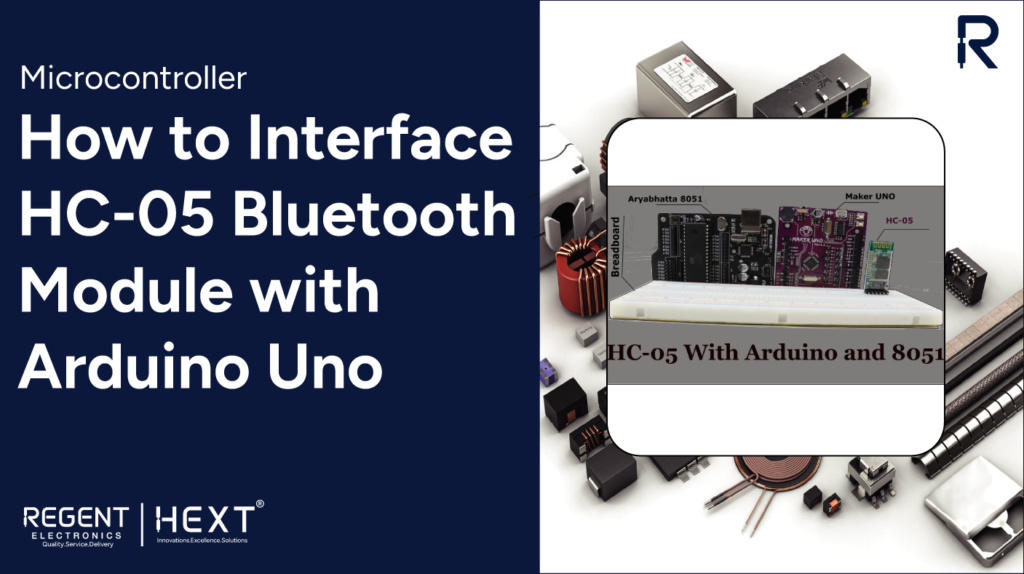
How to Interface HC-05 Bluetooth Module with Arduino Uno | Regent Electronics
If you’re exploring wireless communication for your Arduino projects, the HC-05 Bluetooth module is a perfect starting point. This tutorial by Regent Electronics will walk you through everything you need to know about the HC-05 Bluetooth module, from its basic features to interfacing it with Arduino Uno and even with an 8051 microcontroller.
📡 What is the HC-05 Bluetooth Module?
The HC-05 is a versatile Bluetooth module that supports serial communication (UART), making it easy to connect with microcontrollers like Arduino and 8051. It’s commonly used in IoT, robotics, home automation, and wireless sensor networks.
Key features include:
- Full-duplex communication (send and receive simultaneously)
- Low-power operation
- Frequency-hopping spread spectrum (FHSS) technology
- Secure and reliable communication over short distances
🧠 Why Use HC-05 in IoT Projects?
In short-distance wireless communication, using GSM modules can be overkill—complex and expensive. For local communication (within 10 meters), Bluetooth modules like HC-05 offer a cost-effective and efficient solution.
Whether you’re collecting data from a small system or controlling a robot wirelessly, HC-05 makes the process easier.
📌 HC-05 Pinout and Functionality
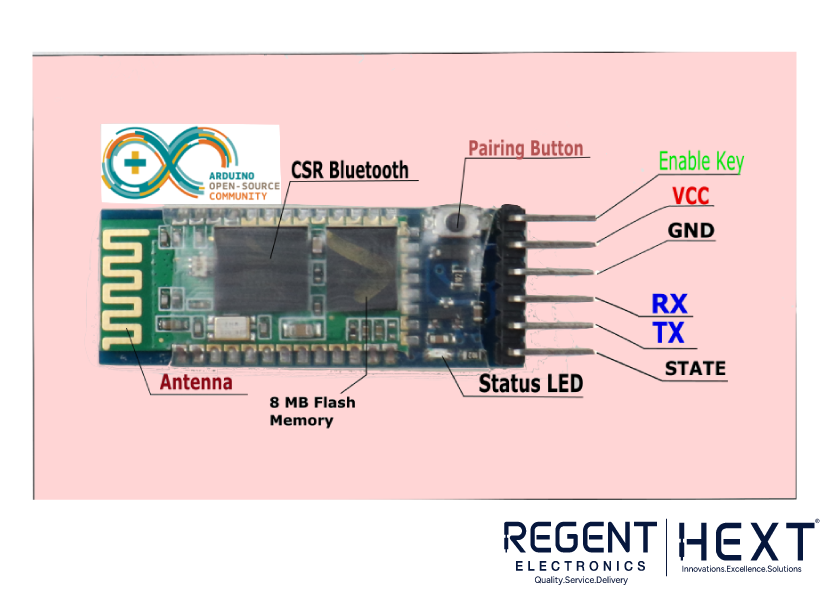
Here’s a breakdown of the HC-05 Bluetooth module’s pin configuration:
Pin | Name | Function |
1 | VCC | Power Supply (3.3V or 5V) |
2 | GND | Ground |
3 | TXD | Transmit Data |
4 | RXD | Receive Data |
5 | STATE | Connection Status Indicator |
6 | EN (KEY) | Mode Selection (Command/Data) |
Two Modes of Operation:
- Data Mode (default): Used for sending/receiving data.
- Command Mode: Used for configuring the module using AT commands.
🔌 Interfacing HC-05 with Arduino Uno
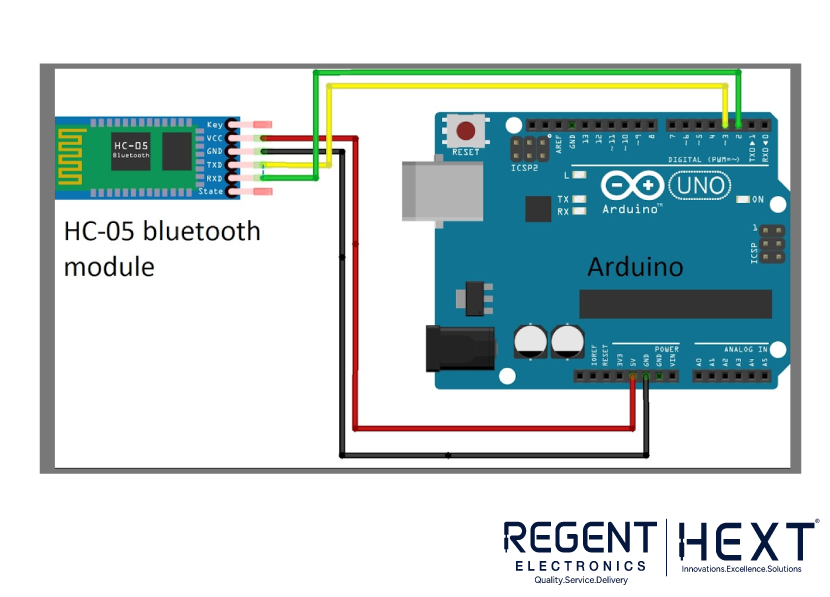
Required Components:
- Arduino Uno
- HC-05 Bluetooth Module
- Jumper Wires
- Arduino IDE
Circuit Connections:
HC-05 Pin | Connects To Arduino Pin |
VCC | 5V |
GND | GND |
TXD | Pin 2 (Software RX) |
RXD | Pin 3 (Software TX) |
⚠️ Use a voltage divider for the RXD pin of HC-05 to avoid over-voltage damage.
💻 Arduino Code to Communicate with HC-05
cpp
CopyEdit
#include <SoftwareSerial.h>
SoftwareSerial BTSerial(2, 3); // RX, TX
void setup() {
Serial.begin(9600);
BTSerial.begin(9600);
BTSerial.println(“Bluetooth Connected”);
}
void loop() {
if (BTSerial.available()) {
char data = BTSerial.read();
Serial.write(data);
}
if (Serial.available()) {
char data = Serial.read();
BTSerial.write(data);
}
}
Upload this code to your Arduino, pair HC-05 with your smartphone (PIN: 1234 or 0000), and start sending commands using a Bluetooth terminal app.
📱 Recommended Android App for Testing
You can use any generic Bluetooth Terminal app available on the Google Play Store. Simply pair the HC-05 with your smartphone and send text commands to communicate with Arduino.
🧩 Interfacing HC-05 with 8051 Microcontroller
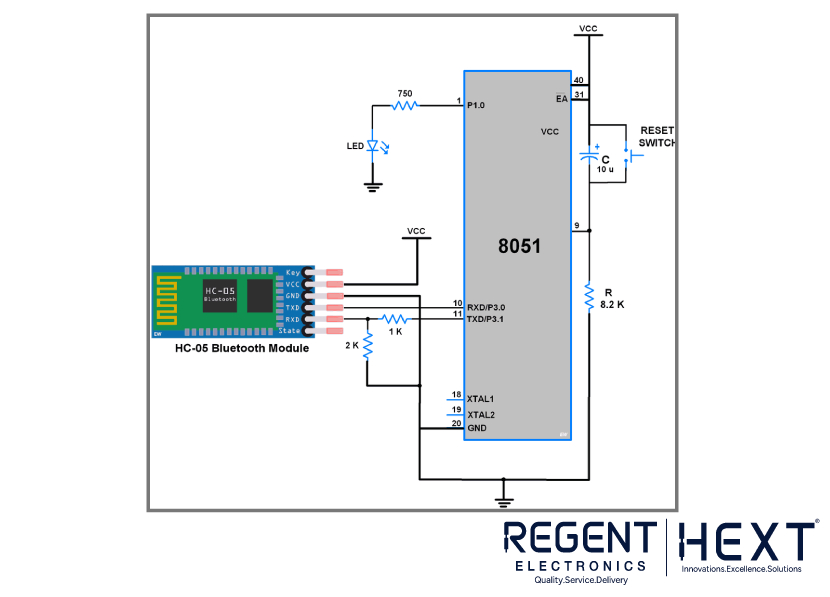
Required Setup:
- 8051 Development Board
- HC-05 Bluetooth Module
- UART Library for 8051
- Power Supply
Sample Code for 8051:
c
CopyEdit
#include <reg51.h>
#include “UART_H_file.h”
sbit LED = P1^0;
void main() {
char data;
UART_Init(); // Initialize UART
P1 = 0;
while (1) {
data = UART_RxChar(); // Receive data
if (data == ‘1’) {
LED = 1;
UART_SendString(“LED_ON”);
} else if (data == ‘2’) {
LED = 0;
UART_SendString(“LED_OFF”);
} else {
UART_SendString(“Invalid Command”);
}
}
}
HC-05 to 8051 Pin Mapping:
HC-05 | 8051 |
VCC | +5V |
GND | GND |
TXD | RX (P3.0) |
RXD | TX (P3.1) |
🧪 Summary
In this tutorial, you learned:
- The basics and features of the HC-05 Bluetooth Module
- How to interface HC-05 with Arduino Uno using SoftwareSerial
- How to send and receive data wirelessly
- Interfacing HC-05 with 8051 microcontroller
Regent Electronics encourages you to try these experiments and build your own Bluetooth-enabled projects. If you have questions, feel free to leave a comment, and our tech team will help you out!