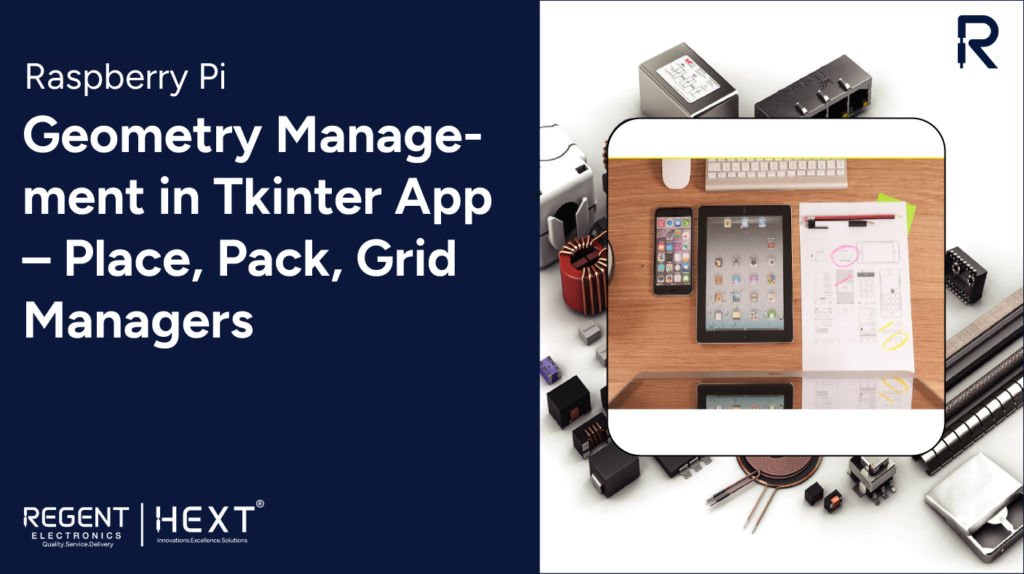
Geometry Management in Tkinter App – Place, Pack, Grid Managers
Introduction
In this tutorial, we will explore how the Grid Manager works in Tkinter and how to effectively use Pack, Place, and Grid Geometry/Layout Managers in your Tkinter application.
Understanding Geometry Managers in Tkinter
When designing a GUI application using Tkinter, managing the layout and positioning of widgets is crucial. Instead of manually specifying width and padding for widgets, we use Tkinter’s built-in geometry managers. These managers help organize widgets efficiently within the application.
Tkinter provides three types of geometry/layout managers:
- Place Geometry Manager
- Pack Geometry Manager
- Grid Geometry Manager
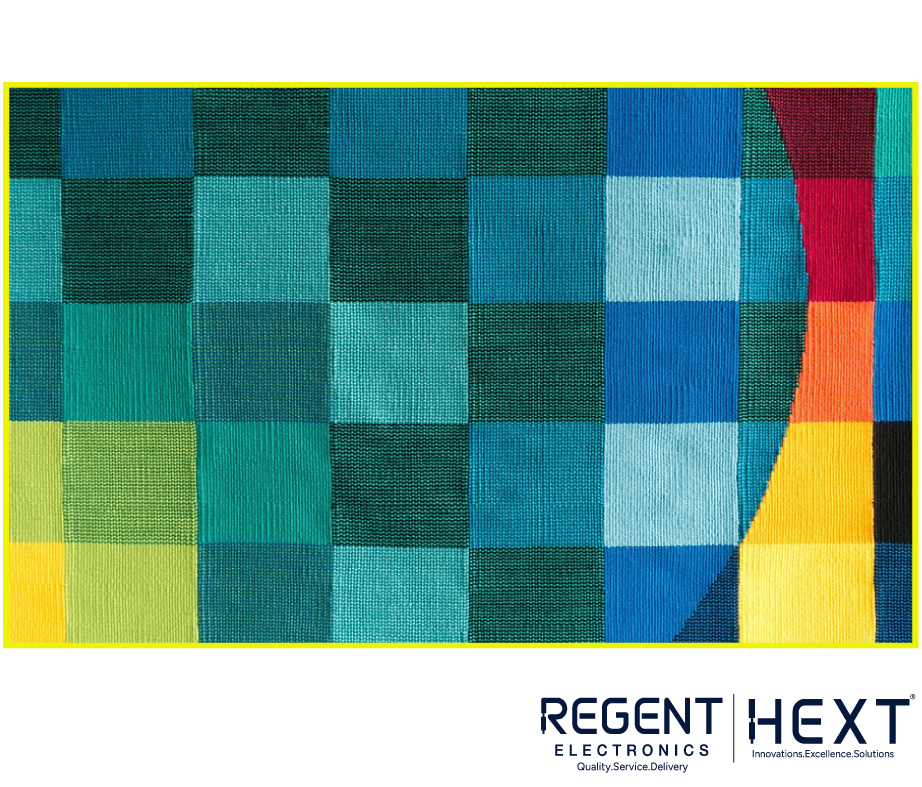
Why Use Geometry Managers in Tkinter?
Geometry Managers allow developers to:
- Control the position and alignment of widgets.
- Organize widgets dynamically based on window size.
- Ensure a responsive UI that adapts to different screen resolutions.
While widget functions can define size and padding, they cannot dictate precise placement. This is where layout managers come into play.
Pack Geometry Manager in Tkinter
The Pack Geometry Manager is one of the simplest ways to manage widgets in Tkinter. It stacks widgets on top of each other and is best suited for simple applications.
Example Code:
from tkinter import ttk
import tkinter as app
app_main_window = app.Tk()
label0 = ttk.Label(app_main_window, text=”Label 1″, background=’Orange’)
label1 = ttk.Label(app_main_window, text=”Label 2″, background=’Green’)
label2 = ttk.Label(app_main_window, text=”Label 3″, background=’Blue’)
label3 = ttk.Label(app_main_window, text=”Label 4″, background=’Magenta’)
label0.pack(fill=’both’, side=’top’, expand=True)
label1.pack(fill=’both’, side=’top’, expand=True)
label2.pack(fill=’both’, side=’top’, expand=True)
label3.pack(fill=’both’, side=’top’, expand=True)
app_main_window.mainloop()
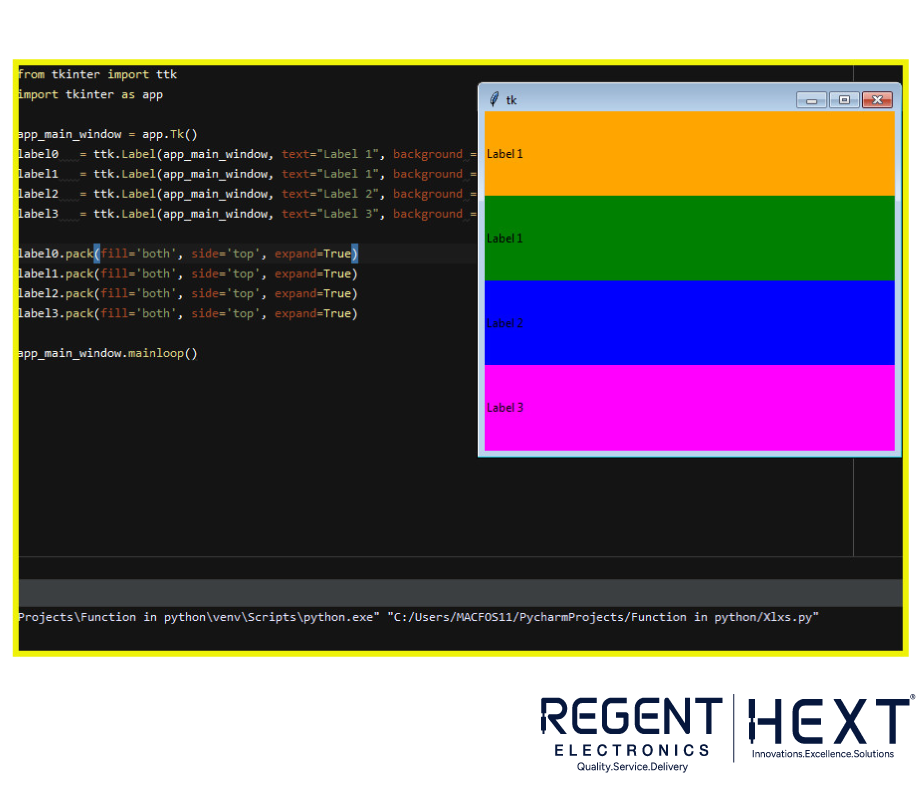
Options Available for Pack Geometry Manager
1. Fill Option in Pack
The fill option allows widgets to stretch within their assigned space. It supports three parameters:
widget.pack(fill=’x’) # Expands horizontally
widget.pack(fill=’y’) # Expands vertically
widget.pack(fill=’both’) # Expands both horizontally and vertically
2. Expand Option in Pack
The expand option determines whether a widget should take up extra space within the layout. It takes Boolean values (True or False).
widget.pack(expand=True) # Expands into unused space
3. Side Option in Pack
The side option allows widgets to be aligned next to each other.
widget.pack(side=’left’) # Aligns to the left
widget.pack(side=’right’) # Aligns to the right
widget.pack(side=’top’) # Default alignment (top)
Example: Placing Widgets Side by Side
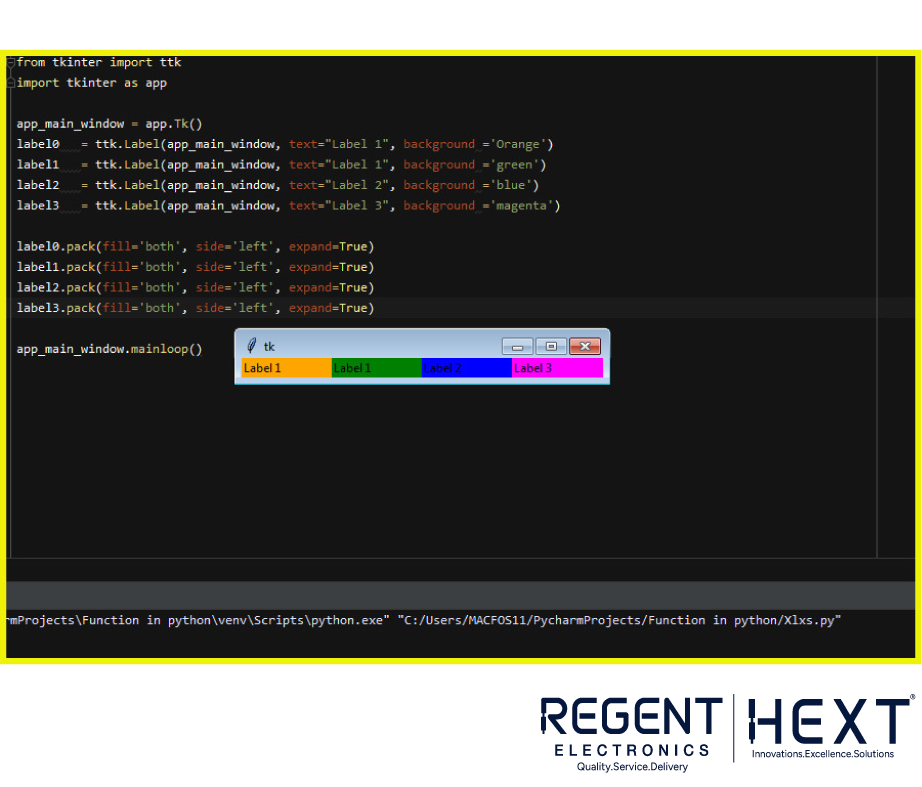
from tkinter import ttk
import tkinter as app
app_main_window = app.Tk()
label0 = ttk.Label(app_main_window, text=”Label 1″, background=’Orange’)
label1 = ttk.Label(app_main_window, text=”Label 2″, background=’Green’)
label2 = ttk.Label(app_main_window, text=”Label 3″, background=’Blue’)
label3 = ttk.Label(app_main_window, text=”Label 4″, background=’Magenta’)
label0.pack(fill=’both’, side=’left’, expand=True)
label1.pack(fill=’both’, side=’left’, expand=True)
label2.pack(fill=’both’, side=’left’, expand=True)
label3.pack(fill=’both’, side=’left’, expand=True)
app_main_window.mainloop()
Conclusion
In this tutorial, we covered the Pack Geometry Manager in Tkinter. In the next tutorial, we will explore the Grid Geometry Manager, which provides more flexibility for aligning widgets in a structured manner.
Stay tuned for more insights on Tkinter layout management! If you have any questions or suggestions, feel free to share them in the comments.